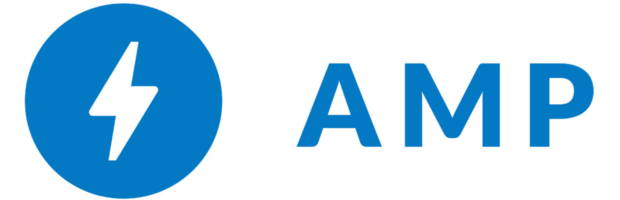
While implementing Google AMP (Accelerated Mobile Pages) for your website, it might occur to you that you might need to update your page, and how would the AMP cache be invalidated/flushed/updated. Google AMP project has an easy solution for this, it’s an API call to the invalidate any URL.
We can use the update-cache request to update and remove content from the Google AMP Cache. Google AMP cache updates content based on the max-age present in the header when the page was last fetched. The update-cache endpoint requires the user to make a signed request using a self generated RSA private key, the public key should be available at a standard location on your website.
I faced the same dilemma, I had read the docs, but couldn’t find any ready-made solution in Perl, so I had to write mine, which I will be sharing with you. Here’s how to get going.
First we need to generate the the private & public keys:
$ openssl genrsa 2048 > private-key.pem $ openssl rsa -in private-key.pem -pubout >public-key.pem $ cp public-key.pem <document-root-of-website>/.well-known/amphtml/apikey.pub
replace <document-root-of-website> with your website’s document root.
Next, here’s the Perl code to which accepts an URL which needs to be invalidated. I have commented the code so its easier to understand.
#!/usr/bin/perl use utf8; use MIME::Base64 qw[encode_base64url]; use Mojo::UserAgent; use Crypt::OpenSSL::RSA; use Mojo::URL; use Mojo::File; ## paths to keys my $path_to_priv_key = 'private-key.pem'; my $ua = Mojo::UserAgent->new; ## get URL from command line argument my $url = shift; unless ( defined($url) && $url ) { die('URL required'); } my $url_obj = Mojo::URL->new($url); ## fetch the JSON containing the caches those need to be invalidated. my $caches = $ua->get('https://cdn.ampproject.org/caches.json')->res->json; unless ( defined($caches) && ref($caches) ) { die('Could not get caches'); } ## load the private key my $priv_key = Mojo::File->new($path_to_priv_key)->slurp; ## create openssl private key instance my $rsa_priv_key = Crypt::OpenSSL::RSA->new_private_key($priv_key); ## select the hashing algo to use, which as specified by Google AMP is SHA-256 $rsa_priv_key->use_sha256_hash(); ## loop through the caches to be invalidated foreach my $cache ( @{ $caches->{caches} } ) { ## build the URL to invalidate my $url_to_sign = sprintf( '/update-cache/c/s/%s%s?amp_action=flush&_ts=%s', $url_obj->host, $url_obj->path, time() ); my $encrypted_sig = $rsa_priv_key->sign($url_to_sign); ## get AMP-style hostname, read more at https://developers.google.com/amp/cache/overview#amp-cache-url-format my $host_amp_style = $url_obj->host; $host_amp_style =~ s/([.-])/($1 eq '.')?'-':'--'/eg; ## URL-safe base64 encode the signature my $sig = encode_base64url($encrypted_sig); ## build API URL to call my $api_url = Mojo::URL->new( sprintf( 'https://%s.%s%s&_url_signature=%s', $host_amp_style, $cache->{updateCacheApiDomainSuffix}, $url_to_sign, $sig ) ); ## make request my $tx = $ua->get($api_url); ## print reponse, you may change this according to your needs print $tx->res->body; }